Basic concepts of Computer Programming Languages
Summary of basic concepts of computer programming languages
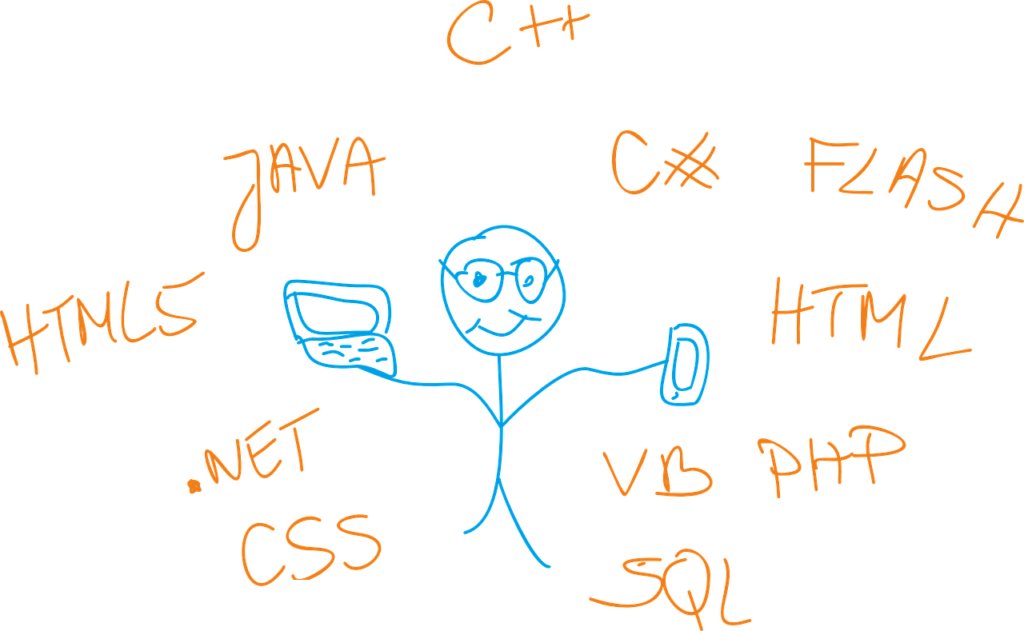
Summary
A computer program is a set of instructions that can be executed by a computer to perform a specific task.
We write programs in a language a computer can understand. Usually, those languages are called programming languages.
Programming languages help us to write the code/program in almost English like language, converts that into a machine level language. Executes them, brings back the result again in a format that we can understand.
Algorithm / Flowcharts
A program always starts with an algorithm. An algorithm is a procedure or steps we follow to solve a problem, where a problem can be mathematical or logical.
Most of the time, we use a flowchart to represent an algorithm. A flow chart is a type of diagram that presents the steps to be followed to solve any given problem. It is easy to read and understand.
A flowchart shows the steps to be followed with different kinds of boxes and arrows to indicate the flow of the process.
The most common blocks used in any flow chart are:
- Terminal block – Strat / End blocks of the program
- Process blocks – Set of operations
- Decision block – which checks the conditions and decides the further path of the process
- Input / Output blocks
Example:
Check whether any given number A is positive or negative
Start
Input the value for A
If A>=0
If yes, the result is Positive
If not, the result value is Negative
Print the output/result
End
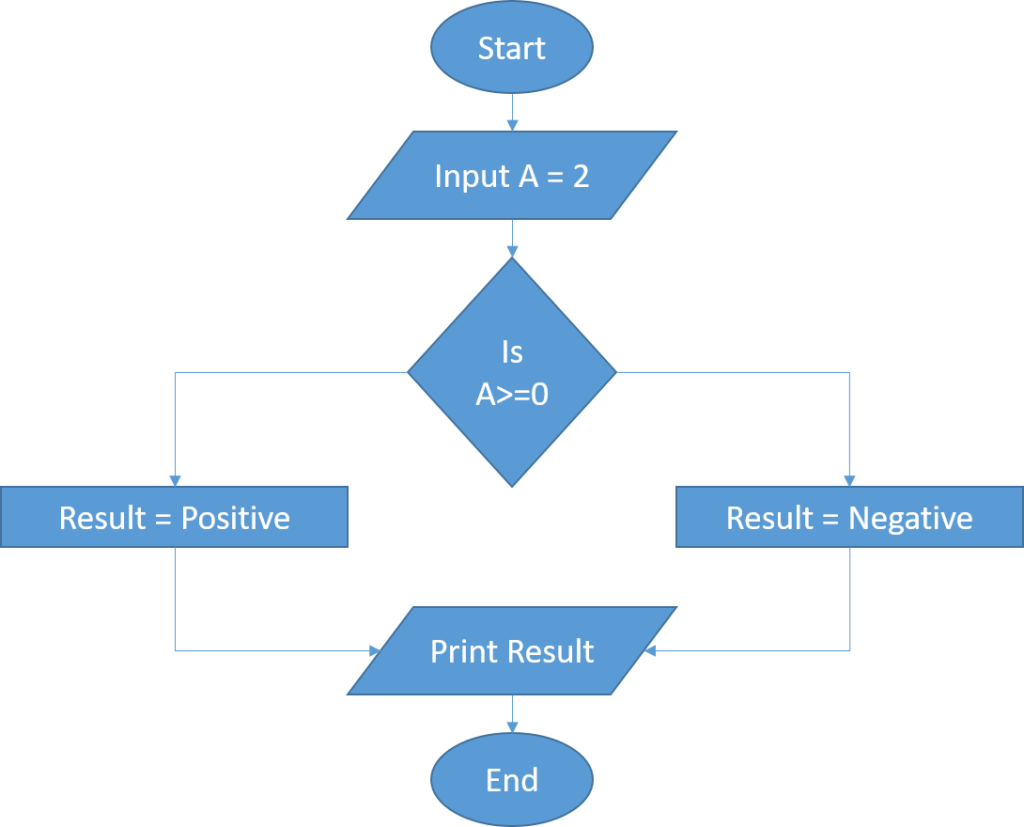
Syntax of programming languages
Just like we humans have hundreds of thousands of languages to speak or write, there are thousands of programming languages to interact with a computer.
Syntax in any programming language is the set of rules to be followed to write a program in that language. The syntax is the grammar of programming languages.
To display “Hello world” in some of the common programming languages, we use different syntaxes, as shown below:
HTML
Hello World!
Basic
PRINT "Hello, world!"
C
#include
int main(void)
{
puts("Hello, world!");
}
C++
#include
int main()
{
std::cout << "Hello, world!";
return 0;
}
C#
using System;
class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Hello, world!");
}
}
Java
import javax.swing.JFrame; //Importing class JFrame
import javax.swing.JLabel; //Importing class JLabel
public class HelloWorld {
public static void main(String[] args) {
JFrame frame = new JFrame(); //Creating frame
frame.setTitle("Hi!"); //Setting title frame
frame.add(new JLabel("Hello, world!"));//Adding text to frame
frame.pack(); //Setting size to smallest
frame.setLocationRelativeTo(null); //Centering frame
frame.setVisible(true); //Showing frame
}
}
JavaScript
document.write('Hello, world!');
jQuery
$("body").append("Hello world!");
The syntax may be different in different languages. But the basic flow of logic used to solve any problem are similar. If you know to write programs in any one language, it’s easy to learn others.
Functions
We can call a function as a mini-program, a set of instructions meant to do a particular task. Functions are named depending on their job.
Example :
Sum(a,b);
Max(a,b,c);
Here Sum and Max are the function names.
Sum(a,b) adds a to be and returns the sum of them as a result.
Similarly Max(a,b,c) retuns the maximum number among a,b and c.
- Functions reduce the repetition of codes within the program
- Increases the readability and understanding of the code
- Easy to modify any program
There are two main types of functions –
- Standard library functions, in-built in the programming languages
- User-defined functions, users create them if they need to run the same task multiple times.
Debugging
Debugging is a process of finding and removing any errors in the code, which are causing the program to behave differently than expected.